Typing Tutor Game
Display text on screen
To display some words on screen, we create some text objects and add them to the screen. Then upate the screen to show the changes by using either display_surf.blit()
and pygame.display.flip()
or update()
.
Font
To draw text on screen, we need to create the font object.
As our game will depend on typing text, we should use a font that has fixed width for each character. We will use Consolas Bold font for our game.
Download the font "Consolas Bold.ttf".
Or get from https://github.com/arynchoong/TypingTutorGame/blob/master/res/Consolas%20Bold.ttf
With the font, we can now set our font object:
class TypingTutor():
def __init__(self):
pygame.init() # initialises Pygame libraries
self.screen = pygame.display.set_mode((WIDTH, HEIGHT))
self.disp_surf = self.init_display()
self.size = WIDTH, HEIGHT
self.running = True if self.disp_surf else False
self.state = START
self.font = pygame.font.Font('Consolas Bold.ttf', 36)
return
Font Colour
Let's set our RGB colour for our welcome screen font:
# R G B
BLACK = ( 0, 0, 0)GREEN = ( 0, 255, 0)
We can now render our text's surface, or in other words generate our image/sprite object.
We'll first create a function that takes the text to display, colour of text and the center position of the text.
def draw_text(self, text, colour, center):
'''Draws text onto diplay surface'''
text_surf = self.font.render(text, True, colour)
And retrieve the rectangle object for our surface:
def draw_text(self, text, colour, center):
'''Draws text onto diplay surface'''
text_surf = self.font.render(text, True, colour)
text_rect = text_surf.get_rect()
To set the position of our object, let us put it at center of out display surface:
def draw_text(self, text, colour, center):
'''Draws text onto diplay surface'''
text_surf = self.font.render(text, True, colour)
text_rect = text_surf.get_rect()
text_rect.center = center
We then have to place it into our draw buffer.
blit
stands for BLock Image Tranfser. It transfers the created image of our text onto our display surface.
def draw_text(self, text, colour, center):
'''Draws text onto diplay surface'''
text_surf = self.font.render(text, True, colour)
text_rect = text_surf.get_rect()
text_rect.center = center
self.disp_surf.blit(text_surf, text_rect)
Then we just can just call the function:
def render(self):
'''Render surface'''
self.disp_surf.fill(BLACK)
# Draw text
self.draw_text("Typing Tutor Game", GREEN, (int(WIDTH/2), int(HEIGHT/2)))
It will be updated to the screen when we flip()
:
# Blit everything to screen
self.screen.blit(self.disp_surf, (0,0))
pygame.display.flip()
Give it a try, you should end up with green text on black background, something like this:
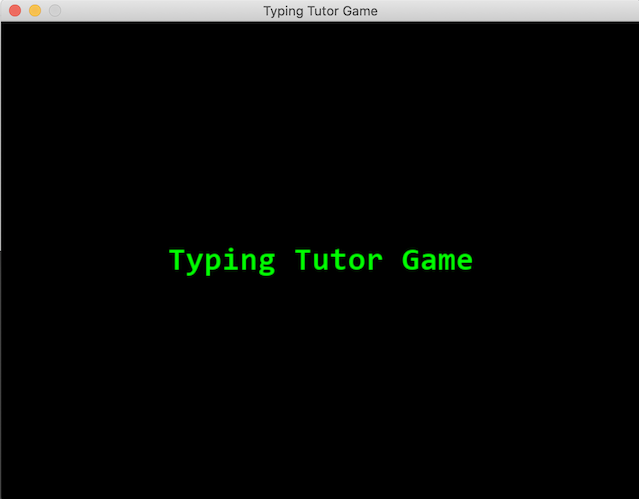
Since we have our game states, we can now check that our events are correctly handled by displaying different text for each event.
def render(self):
'''Render surface'''
self.disp_surf.fill(BLACK)
# set the text for display
if self.state == START:
text = 'Typing Tutorial Game'
message = 'Press space to play.'
else:
text = 'Game Over'
message = 'State: %s' % self.state
# draw the messages
self.draw_text(text, GREEN, (int(WIDTH / 2), int(HEIGHT / 2) - 50))
self.draw_text(message, GREEN, (int(WIDTH / 2), int(HEIGHT / 2) + 50))
# Blit everything to screen
self.screen.blit(self.disp_surf, (0,0))
pygame.display.flip()
return
Test to see if the text changes when you hit the spacebar and only the spacebar.
python3 -m game