Typing Tutor Game
PyGame
Pygame is a cross platform tool to easily develop games in Python.
It is a set of python module that is built on top of the SDL (Simple DirectMedia Layer) library that allows for developers to have low level access to common gaming tools of audio, peripherals and graphics.
For more information about the Pygame module, please see it's wiki page.
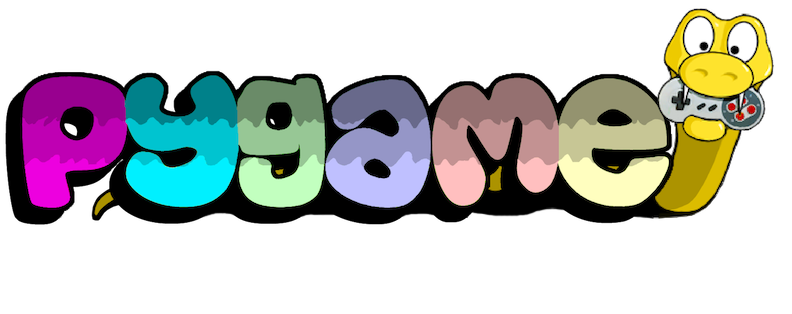
Install
PyGame can be installed using pip. However we need the dev version >= 2.0.0dev for our Typing Tutor example, because I used features only introduced in PyGame 2.0 for getting the keys.
The PyGame version 2.0 is currently still in dev as at 12th March 2020, and so we need to specify the dev version we want to retrieve when we install.
Virtual environment
Remember to set up and activate our virtual environment before we start:
$ python3 -m venv venv
On Windows, run:
$ venv\Scripts\activate.bat
On Unix or MacOS, run:
$ source venv/bin/activate
There are crucial updates in version 2.0 that we will need for our Typing Tutor Game. Namely the key reads.
Installation
Please see: https://github.com/pygame/pygame/releases for the available pre-release versions.
Loook for the latest the latest PyGame Dev version 2.0.0.dev* and install:
(venv) $ pip3 install pygame==2.0.0.dev6
Test your installation by calling the example that comes with the package:
The key here is that it brings up a GUI game.
(venv)$ python3 -m pygame.examples.aliens
PyGame Basics
NOTE: PyGame, at least the GUI part, is not usable (or testable) within jupyter notebooks.
So we will use scripts. Let's start with a script and let's name it 'game.py'.
Load pygame and initialise
To use pygame, lets import the package.
# game.py
import pygame
and we need to initalise pygame to use the library.
We do this in our class, TypingTutor:
class TypingTutor():
def __init__(self):
pygame.init()
if __name__ == "__main__":
game = TypingTutor()
Game display surface area
Next we create our game screen, and call it display surface:
# Dimensions
WIDTH = 640
HEIGHT = 480
class TypingTutor():
def __init__(self):
pygame.init()
self.screen = pygame.display.set_mode((WIDTH, HEIGHT))
self.disp_surf = self.init_display()
self.size = WIDTH, HEIGHT
def init_display(self):
pygame.display.set_caption('Typing Tutor Game')
# Create surface
disp_surf = pygame.Surface(self.screen.get_size())
return disp_surf.convert()
set_caption()
sets the game caption (application title) on the top of the display.
The display surface is our game play surface area, where we load onto the screen where the game runs.
Game loop
And any game will require user input in some form or another, let us now add the codes to check for trigger to close the game. (user clicking the red cross icon on top corner of our display surface)
self.size = WIDTH, HEIGHT
self.running = True if self.disp_surf else False
def execute(self):
# Event loop
while self.running:
self.check_events()
return
def check_events(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.running = False
return
return
Now we just need to execute the codes.
if __name__ == "__main__":
game = TypingTutor()
game.execute()
The codes set a flag called running
and check all the events for the game, if it gets the event pygame.QUIT
, it exits the loop.
Test it
Test your codes by calling the module from console:
And hit the red cross button, it should exit our game and quit. Top left for Macs and Unix, top right for Windows.
python3 -m game
This is the quick show of what is required to use PyGame.
Next we need to figure out how we want our game to work.