PyGame Basics
Animate graphic object
Let's add an image to our text only screen.
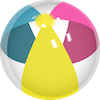
To load an image, we use pygame.image.load()
, and pass in the file path. The pygame.image.load()
function returns a Surface with the ball surface data.
To can manipulate the image by retrieving the rectangular object of the image, Rect
.
class Game():
def __init__(self):
pygame.init() # initialises Pygame libraries
self.display = pygame.display.set_mode((WIDTH, HEIGHT))
self.disp_surf = self.init_display()
self.size = WIDTH, HEIGHT
self.running = True if self.disp_surf else False
self.font = pygame.font.Font(pygame.font.get_default_font(), 36)
self.ball = pygame.image.load("ball.png")
self.ballrect = self.ball.get_rect()
self.speed = [2, 2]
return
We want to display the ball animation.
Let's create render()
.
Here we want to animate our beach ball.
def render(self):
'''Render surface'''
self.disp_surf.fill(BLACK)
# Draw text
self.draw_text("PyGame Basics", GREEN, (int(WIDTH/2), int(HEIGHT/2)))
self.display.blit(self.disp_surf, (0,0))
# setup ball
self.ballrect = self.ballrect.move(self.speed)
self.display.blit(self.ball, self.ballrect)
# update display
pygame.display.flip()
# update speed
if self.ballrect.left < 0 or self.ballrect.right > WIDTH:
self.speed[0] = -self.speed[0]
if self.ballrect.top < 0 or self.ballrect.bottom > HEIGHT:
self.speed[1] = -self.speed[1]
return
We use the Rect.move()
to change the position of the ball Surface by the speed
. Which means it move (2,2)
pixels on every cycle on its horizontal and vertical axis.
We then draw the ball with blit()
Pygame manages the display with a second buffer, and to update the current buffer with the one we just drawn we call pygame.display.flip()
.
After drawing, we update the speed of the ball if it moves off screen. To change the direction of movement, we invert the speed in that direction.
Test the codes. We should have a diagonally moving ball, bouncing about.