PyGame
Pygame is a cross platform tool to easily develop games in Python.
It is a set of python module that is built on top of the SDL (Simple DirectMedia Layer) library that allows for developers to have low level access to common gaming tools of audio, peripherals and graphics.
For more information about the Pygame module, please see it's wiki page.
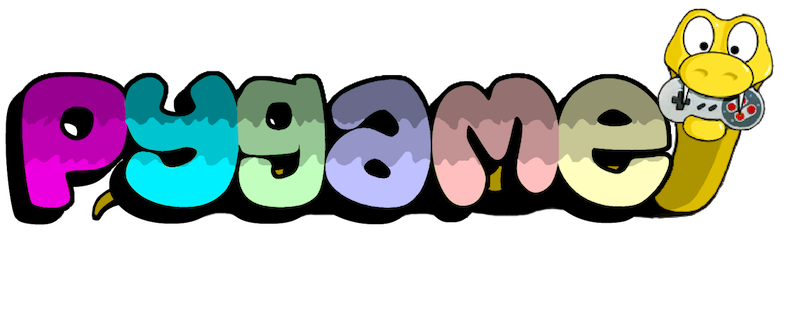
Install
PyGame can be installed using pip.
Virtual environment
Remember to set up and activate our virtual environment before we start:
$ python3 -m venv venv
On Windows, run:
$ venv\Scripts\activate.bat
On Unix or MacOS, run:
$ source venv/bin/activate
Installation
If you would like to install a specific version, please see: https://github.com/pygame/pygame/releases.
For Mac users on Cantalina OS, you will need to install the 2.0.x.dev version.
(venv) $ pip3 install pygame==2.0.0.dev6
Test your installation by calling the example that comes with the package:
The key here is that it brings up a GUI game.
(venv)$ python3 -m pygame.examples.aliens
PyGame Basics
NOTE: PyGame, at least the GUI part, is not usable (or testable) within jupyter notebooks.
So we will use scripts. Let's start with a script and let's name it 'game.py'.
Load pygame and initialise
To use pygame, lets import the package.
# game.py
import pygame
and we need to initalise pygame to use the library.
We do this in our class, Game:
class Game():
def __init__(self):
pygame.init()
if __name__ == "__main__":
game = Game()
Game display surface area
Next we create our game screen, and call it display surface:
# Dimensions
WIDTH = 640
HEIGHT = 480
class Game():
def __init__(self):
pygame.init()
self.size = WIDTH, HEIGHT
self.display = pygame.display.set_mode(self.size)
self.disp_surf = self.init_display()
def init_display(self):
# Create surface
disp_surf = pygame.Surface(self.size)
return disp_surf.convert()
The display surface is our game play surface area, where we load onto the screen when the game runs.
Events
And any game will require user input in some form or another, let us now add the codes to check for trigger to close the game. (user clicking the red cross icon on top corner of our display surface)
First we add a running
flag to only loop when we have successfully initialised our display surface.
class Game():
def __init__(self):
pygame.init()
self.size = WIDTH, HEIGHT
self.display = pygame.display.set_mode(self.size)
self.disp_surf = self.init_display()
self.running = True if self.disp_surf else False
We then add an execute
function to perform the loop.
class Game():
def __init__(self):
pygame.init()
self.size = WIDTH, HEIGHT
self.display = pygame.display.set_mode(self.size)
self.disp_surf = self.init_display()
self.running = True if self.disp_surf else False
def execute(self):
# Event loop
while self.running:
self.check_events()
return
def check_events(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.running = False
return
return
The check_events()
function will take care of checking if the user has hit the red cross, and quit the game when that happens.
The codes check all the events for the game, if it gets the event pygame.QUIT
, it exits the loop.
Now we just need to execute the codes.
if __name__ == "__main__":
game = Game()
game.execute()
Test it
Test your codes by calling the module from console:
And hit the red cross button, it should exit our game and quit. Top left for Macs and Unix, top right for Windows.
python3 -m game
This is the quick show of what is required to use PyGame.